When a client installs your WebCommander plugin, the platform will call the root URL of your site and render the page through an iframe as the plugin’s manage page. To ensure your plugin works seamlessly within the WebCommander ecosystem, it’s recommended to develop your root URL as a page that provides all the necessary plugin configurations. Follow WebCommander’s guidelines and best practices to create a user-friendly and efficient root URL.
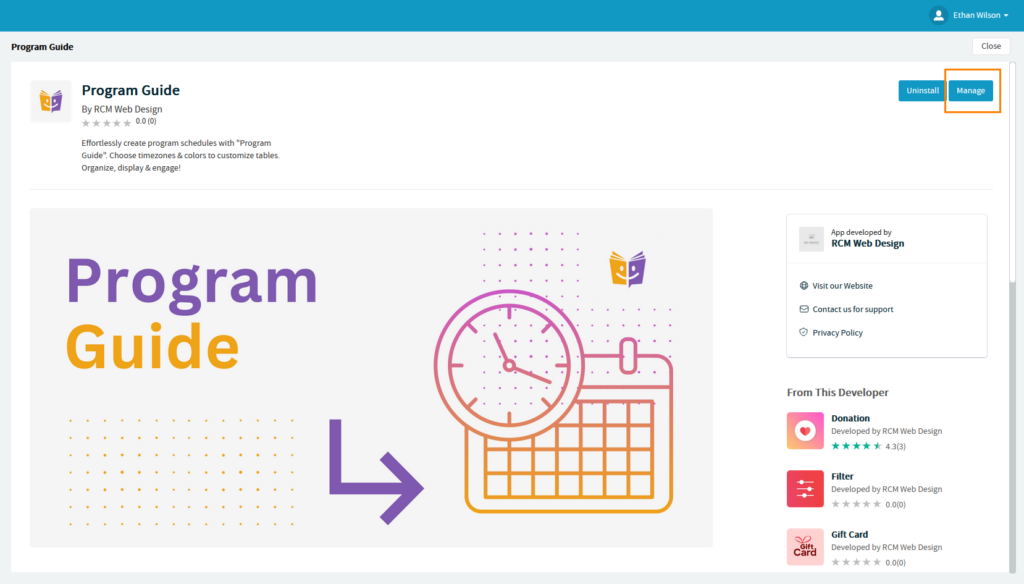
The screenshots above show the manage page of the Clubeez plugin, which is rendered through an iframe inside the WebCommander admin. You don’t need to worry about how the forms on this page communicate with WebCommander site data, as this is your plugin’s page and it can directly communicate with the plugin database. You can use the sample request below as a reference:
cURL
PHP – cURL
Python – http.client
Java – Unirest
C# – RestSharp
curl --location 'https://yourapp.com?uuid=F8A3-A88E-C6EF-B1CB×tamp=1683788339&hmac=CIaGf7Ely0ykfBOi94hEPUAacyaHmjB4oZHc0JMVLMM%3D'
<?php $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://yourapp.com?uuid=F8A3-A88E-C6EF-B1CB×tamp=1683788339&hmac=CIaGf7Ely0ykfBOi94hEPUAacyaHmjB4oZHc0JMVLMM=', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'GET', )); $response = curl_exec($curl); curl_close($curl); echo $response;
import http.client conn = http.client.HTTPSConnection("yourapp.com") payload = '' headers = {} conn.request("GET", "/?uuid=F8A3-A88E-C6EF-B1CB×tamp=1683788339&hmac=CIaGf7Ely0ykfBOi94hEPUAacyaHmjB4oZHc0JMVLMM=", payload, headers) res = conn.getresponse() data = res.read() print(data.decode("utf-8"))
Unirest.setTimeouts(0, 0); HttpResponse<String> response = Unirest.get("https://yourapp.com?uuid=F8A3-A88E-C6EF-B1CB×tamp=1683788339&hmac=CIaGf7Ely0ykfBOi94hEPUAacyaHmjB4oZHc0JMVLMM%3D") .asString();
var options = new RestClientOptions("") { MaxTimeout = -1, }; var client = new RestClient(options); var request = new RestRequest("https://yourapp.com?uuid=F8A3-A88E-C6EF-B1CB×tamp=1683788339&hmac=CIaGf7Ely0ykfBOi94hEPUAacyaHmjB4oZHc0JMVLMM=", Method.Get); RestResponse response = await client.ExecuteAsync(request); Console.WriteLine(response.Content);
Request Details
Request URL: https://yourapp.com/
Request Method: GET
Request Parameter
Name | Type | Description |
---|---|---|
uuid | String | Plugins UUID |
timestamp | String | The time of the request or the current time |
hmac | String | The encryption key we found from the installation process contains encrypted data to validate requests |